目的
タイトル/メッセージ/ネガポジボタンを有したダイアログを作りたい。
AlertDialogでいいじゃん!って感じだけど、デザインと異なるっていう理由でカスタム汎用的なカスタムDialogFragmentを作る羽目に。
ダイアログクラスのベースはこの記事で書いたやつ。
実装
レイアウト
styleとか使ってるけど、適宜修正してもらえればとw
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/background"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/dialogBackground"
tools:ignore="SpUsage">
<LinearLayout
android:id="@+id/dialogTopLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_margin="@dimen/margin_dialog"
android:background="@drawable/bg_white_round"
android:orientation="vertical"
android:padding="@dimen/padding_dialog">
<TextView
android:id="@+id/titleTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="@dimen/margin_dialog_contents_vertical"
android:textColor="@color/baseBlack"
android:textSize="@dimen/font_size_17dp"
android:textStyle="bold"
tools:text="タイトル" />
<TextView
android:id="@+id/messageTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="@dimen/margin_dialog_contents_vertical"
android:textColor="@color/black600"
android:textSize="@dimen/font_size_14dp"
android:textStyle="bold"
tools:text="メッセージ" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="end"
android:orientation="horizontal">
<TextView
android:id="@+id/negativeButton"
style="@style/button_small_primary"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="@dimen/padding_dialog"
android:visibility="gone"
tools:text="@string/close"
tools:visibility="visible" />
<TextView
android:id="@+id/positiveButton"
style="@style/button_small_primary"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/close" />
</LinearLayout>
</LinearLayout>
</FrameLayout>
ダイアログクラス
//
class SimpleDialogFragment : FullScreenDialogBaseFragment() {
companion object {
const val tag = "SimpleDialogFragment"
/**
* requestCodeをクリック時に返します。
* positiveLabelがnullの場合は「閉じる」ボタンを表示し、他のものがnullの場合は非表示にします。
*/
@JvmStatic
fun newInstance(
requestCode: Int,
title: String? = null, msg: String? = null,
positiveLabel: String? = null, negativeLabel: String? = null
) = SimpleDialogFragment().apply {
arguments = Bundle().apply {
putInt(SimpleDialogFragment::requestCode.name, requestCode)
putString(SimpleDialogFragment::title.name, title)
putString(SimpleDialogFragment::msg.name, msg)
putString(SimpleDialogFragment::positiveLabel.name, positiveLabel)
putString(SimpleDialogFragment::negativeLabel.name, negativeLabel)
}
}
}
interface OnSimpleDialogClickListener {
fun onSimpleDialogPositiveClick(requestCode: Int)
fun onSimpleDialogNegativeClick(requestCode: Int)
}
var listener: OnSimpleDialogClickListener? = null
private var requestCode: Int by Arguments()
private var title: String? by Arguments()
private var msg: String? by Arguments()
private var positiveLabel: String? by Arguments()
private var negativeLabel: String? by Arguments()
override fun onAttach(context: Context) {
super.onAttach(context)
// Fragment優先
val fragment = targetFragment
if (fragment is OnSimpleDialogClickListener) {
listener = fragment
} else if (context is OnSimpleDialogClickListener) {
listener = context
}
}
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
super.onCreateView(inflater, container, savedInstanceState)
return inflater.inflate(R.layout.dialog_simple, container, false)
}
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
dialogTopLayout.setOnTouchListener { _, _ -> true }
// タイトル
titleTextView.text = title
// メッセージ
messageTextView.text = msg
// ポジティブボタン
if (!positiveLabel.isNullOrEmpty()) {
positiveButton.text = positiveLabel
}
// ネガティブボタン
if (!negativeLabel.isNullOrEmpty()) {
negativeButton.text = negativeLabel
negativeButton.visibility = View.VISIBLE
negativeButton.setOnClickListener {
listener?.onSimpleDialogNegativeClick(requestCode)
dismiss()
}
}
positiveButton.setOnClickListener {
listener?.onSimpleDialogPositiveClick(requestCode)
dismiss()
}
}
}
by Arguments()とかあまり見慣れないだろうもの出てくるけど、こちらの記事で書いてます。
使い方の一例
SimpleDialogFragment.newInstance(
requestCode = 100,
title = "タイトルですよ",
msg = "メッセージですよ",
positiveLabel = "ポジラベル",
negativeLabel = "ネガラベル"
).show(supportFragmentManager, SimpleDialogFragment.tag)
見た目
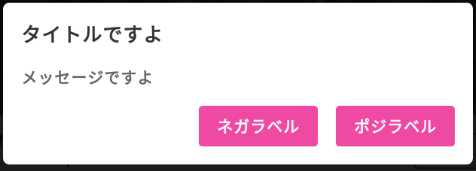